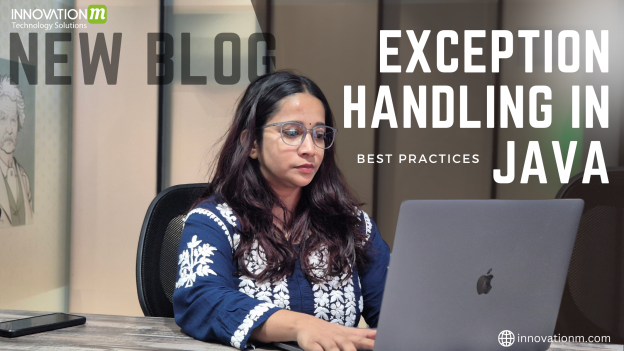
Exception Handling in Java: Best Practices
Exception handling is a crucial aspect of Java programming, particularly when designing APIs and ensuring robust application behavior. This article outlines best practices for handling exceptions in Java, using Spring Boot for RESTful APIs, and emphasizes the importance of providing clear, helpful error messages to consumers. Here’s a comprehensive look into exception handling, using references Continue Reading »