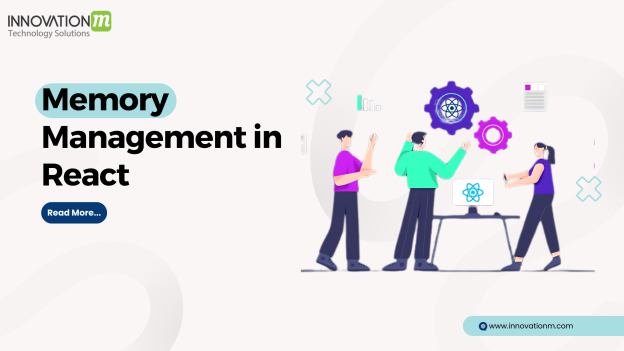
Memory Management in React: Best Practices and Techniques
Memory management is a critical aspect of web development, ensuring that applications run smoothly and efficiently without consuming excessive system resources. In the context of React, a popular JavaScript library for building user interfaces, effective memory management can significantly enhance the performance and user experience of your applications. This blog post delves into the key Continue Reading »